Table Of Content

Next, we ventured into expert-level territory, introducing alternative approaches such as the Composite and State patterns. We provided code examples for these patterns, discussed their advantages and disadvantages, and provided recommendations for their use. This pattern ensures that a class only has one instance and provides a global point of access to it. In conclusion, while design patterns in Java are powerful tools, they must be used judiciously and appropriately. Understanding the potential issues and how to avoid them will help you use design patterns more effectively in your Java projects. We define three subsystem classes, SubsystemA, SubsystemB, and SubsystemC, each with their respective operation methods.
Decorator Pattern
The idea is to keep the service layer separate from the data access layer. The singleton pattern restricts the instantiation of a Class and ensures that only one instance of the class exists in the Java Virtual Machine. The implementation of the singleton pattern has always been a controversial topic among developers. A design pattern is a well-described solution to a common software problem.
Delving into Observer and Decorator Patterns
Lets you define a family of algorithms, put each of them into a separate class, and make their objects interchangeable. The pattern allows you to produce different types and representations of an object using the same construction code. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering. It is a description or model for problem-solving that may be applied in a variety of contexts. Prior to React 16.8, managing state within functional components was a challenge. You had to rely on class components or techniques like render props and higher-order components (HOCs) to achieve stateful behavior.
The Template Method Pattern
It's useful when you want to share common behavior between classes with different implementations. There are many design patterns, each with its own purpose and usage. Creational patterns are used to create objects and manage their creation. Structural patterns are used to organize code into larger structures, making it easier to manage and modify. Behavioral patterns are used to manage communication between objects and control the flow of data. Java design patterns are divided into three categories - creational, structural, and behavioral design patterns.
8 Template Method
180 Core Java Interview Questions and Answers [2024] - Simplilearn
180 Core Java Interview Questions and Answers .
Posted: Wed, 10 Apr 2024 07:00:00 GMT [source]
In particular, they can provide a great deal of flexibility about which objects are created, how those objects are created, and how they are initialized. So in behavioral design we are trying to deal with algorithms and the assignment of responsibilities between objects. The Mediator pattern defines an object that encapsulates how a set of objects interact. It promotes loose coupling by keeping objects from referring to each other explicitly and allows their interaction to be changed independently.
Recommended if you're interested in Software Development
In object-oriented programming, we typically use a lot of inheritance. If somebody wants to create a male person, they invoke the getPerson() method on the PersonFactory with a gender argument of "M". Similarly, you can create a female person by invoking the getPerson() method on the PersonFactory with a gender argument of "F". Whenever a new chess game is started, for example, in any of the numerous online Chess portals, this initialized instance is merely copied or cloned.
9 Clean Code Patterns I wish I knew earlier by Martin Thoma - Towards Data Science
9 Clean Code Patterns I wish I knew earlier by Martin Thoma.
Posted: Sat, 16 Oct 2021 07:00:00 GMT [source]
Pattern 2: Higher-Order Components (HOCs)
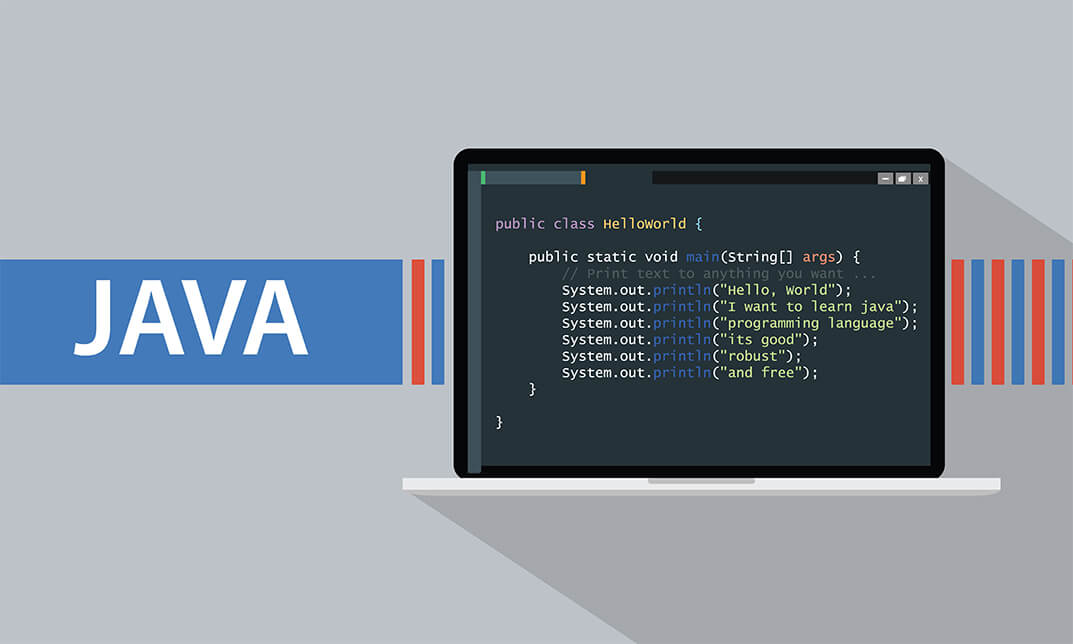
The Strategy pattern defines a family of algorithms, encapsulates each one, and makes them interchangeable. It's useful when you have multiple ways to solve a problem and want to choose an algorithm at runtime. Sometimes, developers force the use of design patterns where they’re not needed, leading to unnecessary complexity.
Behavioral Patterns
By studying and implementing these patterns in your projects, you can improve your skills as a developer and make your software more adaptable to change. Don't forget to practice implementing these patterns in your projects to get a better grasp of their usage. The Factory pattern provides an interface for creating objects in a super class, allowing subclasses to decide which class to instantiate. It promotes loose coupling by eliminating the need for clients to know about the concrete classes. In the context of software development, a design pattern is a reusable solution that can be applied to common problems encountered in software design. They represent best practices evolved over a period of time and are named in a way that can convey their intent.
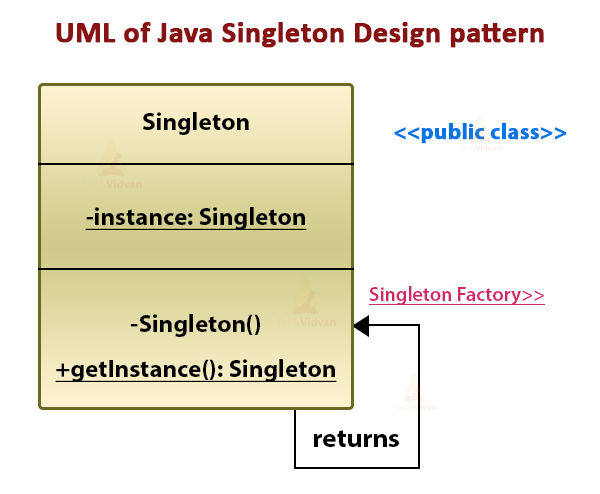
Top Creational Design Patterns With Real Examples in Java
Lets you ensure that a class has only one instance, while providing a global access point to this instance. Null Object Method is a Behavioral Design Pattern, it is used to handle the absence of a valid object by providing an object that does nothing or provides default behavior. State Method is a Behavioral Design Pattern, it allows an object to alter its behavior when its internal state changes.
To become a professional software developer, you must know at least some popular solutions (i.e. design patterns) to the coding problems. Defines the skeleton of an algorithm in the superclass but lets subclasses override specific steps of the algorithm without changing its structure. AccountFactory is a class that implements the Factory design pattern. It uses the accountType to create the corresponding type of BankAccount. Now let's explore the ways to implement the Factory design pattern together. From the class diagram, coders can easily see that the implementation for Factory is extremely straightforward.
In case you need to define an additional speed level, just add in a new class that implements the SpeedLevel interface and implement its rotate method. You have a set of elements arranged in a collection, and you want to sequentially access those elements. A good example of an Iterator is a TV remote, which has the “next” and “previous” buttons to surf TV channels. Pressing the “next” button takes me one channel in the forward direction, and pressing the “previous” button takes me one channel in the backward direction. The drawback of this approach is the complexity involved in creating objects.
In this pattern, the request is sent to the invoker and the invoker passes it to the encapsulated command object. The command object passes the request to the appropriate method of receiver to perform the specific action. Behavioral patterns provide a solution for better interaction between objects and how to provide loose-coupling and flexibility to extend easily. We must use the design patterns during the analysis and requirement phase of SDLC(Software Development Life Cycle). Render Props offer a unique way to achieve customization in React components. They allow you to pass down rendering logic as a prop to a component, giving the consuming component control over how its data is displayed.
No comments:
Post a Comment